You have a bunch of strings defined in Localizable.strings.
"FooString" = "Have a Foo.";
"BarString" = "Anyone for Bar?";
"BazString" = "Please pass the Baz.";
You don't want to pass a string literal to NSLocalizedString()
because (1) it's easy to make a typo, and (2) it's tedious since autocomplete can't assist.
NSArray *strings = @[
NSLocalizedString(@"FooString", nil),
NSLocalizedString(@"BarStrung", nil), // Oops.
NSLocalizedString(@"BazString", nil)
];
You create a bunch of string constants instead.
static NSString *FooString = @"FooString";
static NSString *BarString = @"BarString";
static NSString *BazString = @"BazString";
Now you get autocomplete, and if you fat-finger anyway, the compiler flags it and Xcode is eager to fix it for you.
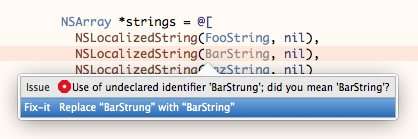
But you still have to type
static NSString *BlaBlaBlaBla = @"BlaBlaBlaBla";
when a string is added to Localized.strings, which is still tedious and typo-prone.
Fortunately, there is no tedious task that can't be simultaneously shortened and obfuscated by the judicious application of preprocessor macros.
#pragma mark Localizable string keys
#define _STRINGIFY(s) #s
#define STRINGIFY(s) _STRINGIFY(s)
#define $(x) *x = @STRINGIFY(x)
static NSString $(FooString);
static NSString $(BarString);
static NSString $(BazString);
... etc ...
#undef _STRINGIFY
#undef STRINGIF
#undef $
And yes, I probably could have written a build script to autogenerate the string definitions from Localizable.strings in less time than it took to write this.